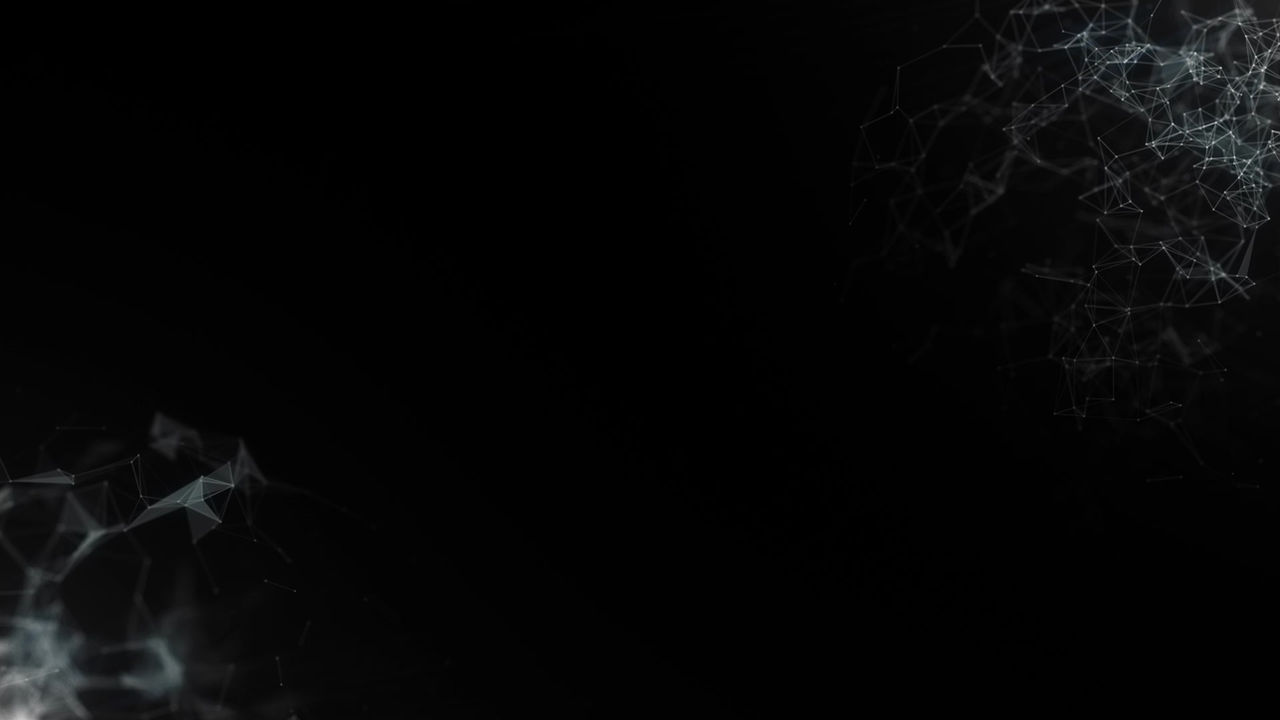
RTS Mobile Game WIth UNity
Part 1:Pathfinding
I like RTS games such as starcraft 2,Dawn of War and Homeworld(Gearbox recently remastered this! hurray).So I wanted to learn the techniques and face the challenges on making an RTS game on mobile since I havent played too many RTS games on mobile.
In this first part I wanted to concentrate on the pathfinding system since it is essential that this is optimized well as almost every unit in the game uses it in some way so its worth it to get it right.There are ofcourse pre made systems out there such as the A* pathfinding project but I wanted to implement it on my own for 2 reasons.Firstly I wanted to face the challenge of making a system from scratch and learning about it as I implement it and secondly it is easier to optimize an in house solution rather than a system developed by somone else as each RTS game is diffrent in its own way and would have diffrent needs from the pathfinding algorithm.
To start with I implemented my own version of the A* algorithm.Here is a breakdown of my approach
1)A grid of nodes is created in the beginning depending on map size and size of each node.
2)Each unit requests a Manager to give it a path from a starting vector point to and ending vector point.
3)The manager then adds this request to the queue and requests the pathfinding script to evaluate the next item in the queue.
4)The pathfiding algorithm calculates the smallest neighbour of the starting node based on their g cost and h cost and does a similar process for each subseqent neighbour until it reaches the target node.
5) The path returned is then simplified by removing the nodes that do not change the direction of the unit and this path is returned to the units call back function.
6)The path is then followed by the unit using a path following algorithm
The biggest optimization in the algorithm that I made was to use a heap to store and sort the neighbours.This helps a lot as it reduced the computation time by a good margin.The picture below shows the profilers statistics on the pathfinding which shows only a few occasinal small spikes for calulating path.
However there are still some areas that need to be worked on:
1)Dynamic group movement for when multiple units move as a group
2)Dynamic obstacle avoidance
3)Currently the algorithm works well for user movement commands but needs to be tweaked for continous pathfinding for use in FSM or for moving targets
I will be working on solving the other problems as I move forward with the project and will start testing it on my One Plus X phone to see its performance on Android platform.

Part 1:More Pathfinding and UI
To improve on the pathfinding and give it some polish I decided to add some UI elements and lay out groundworks for implemeting the UI interfaces for the movement.I refactored the code for how the mouse handled the movement for the units by using the classic "Command Pattern" which I learned from the excellent book Game Programming Patterns by Robert Nystrom (highly recommended for any industry gameplay prorgammer).This pattern now has the flexibility to allow me to stream the recieved input as commands for movement.
Through impementing this I was able to implement group movement in an easier manner.Now units will not clump up and have target destinations based on points around the main destination point for the group.
I also added a Sound Manager implemented as Singleton which handles the various voice acknowledgements(from the wraith in starcraft!!) and the main background music(from the game Homeworld).
Next I will be further tweaking the pathfinding for dynamic obstacle and implement the attack function so we can finaly shoot things down!.
Part 2:Object Recycling
One important problem that cannot be ignored is the memory management of Unity (or C# garbage collection).Functions such as Instantiating an object and destroying an object are quite heavy.Not only does this include fetching memory but also deallocating it ,both of which are costly and in case of things such as bullets (imagine multiple ships shooting at diffrent enemies) would absolutely murder the frame-rate.Thats no fun at all!!
So for this I created an Object Recycler script that acts as an object pool(you can see it recycling through bullet objects on the left of the video).This script basicaly loads a list of objects in the beginning and then allocates objects from this list to the various GameObjects in the game.To test this I used it on the bullets but it can be used for any GameObject.
Moving forward this will be used for the various available units as well.This is a relatively easy task (especialy when you use librares like System.Linq) but still quite essential if this game has to perform on Mobile.